mirror of
https://github.com/robertkrimen/otto.git
synced 2024-05-31 14:13:44 +08:00
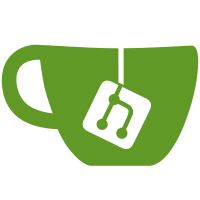
Enable more linters, address the issues and do a major naming refactor to use golang lower camelCase identifiers for types, functions, methods and variable names. Also: * Clean up inline generation so it doesn't rely on temporary variables. * Remove unused functions generated by inline.pl.
47 lines
899 B
Go
47 lines
899 B
Go
package otto
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"strings"
|
|
)
|
|
|
|
func formatForConsole(argumentList []Value) string {
|
|
output := []string{}
|
|
for _, argument := range argumentList {
|
|
output = append(output, fmt.Sprintf("%v", argument))
|
|
}
|
|
return strings.Join(output, " ")
|
|
}
|
|
|
|
func builtinConsoleLog(call FunctionCall) Value {
|
|
fmt.Fprintln(os.Stdout, formatForConsole(call.ArgumentList))
|
|
return Value{}
|
|
}
|
|
|
|
func builtinConsoleError(call FunctionCall) Value {
|
|
fmt.Fprintln(os.Stdout, formatForConsole(call.ArgumentList))
|
|
return Value{}
|
|
}
|
|
|
|
// Nothing happens.
|
|
func builtinConsoleDir(call FunctionCall) Value {
|
|
return Value{}
|
|
}
|
|
|
|
func builtinConsoleTime(call FunctionCall) Value {
|
|
return Value{}
|
|
}
|
|
|
|
func builtinConsoleTimeEnd(call FunctionCall) Value {
|
|
return Value{}
|
|
}
|
|
|
|
func builtinConsoleTrace(call FunctionCall) Value {
|
|
return Value{}
|
|
}
|
|
|
|
func builtinConsoleAssert(call FunctionCall) Value {
|
|
return Value{}
|
|
}
|